developer-sam.de
Hi, I’m Developer Sam and this is my public online-space. I write mainly about software development with a focus on testable database development and code quality, but am not limiting myself to these topics, because I’m a whole person.
There are times where you are so occupied that you forget important things.
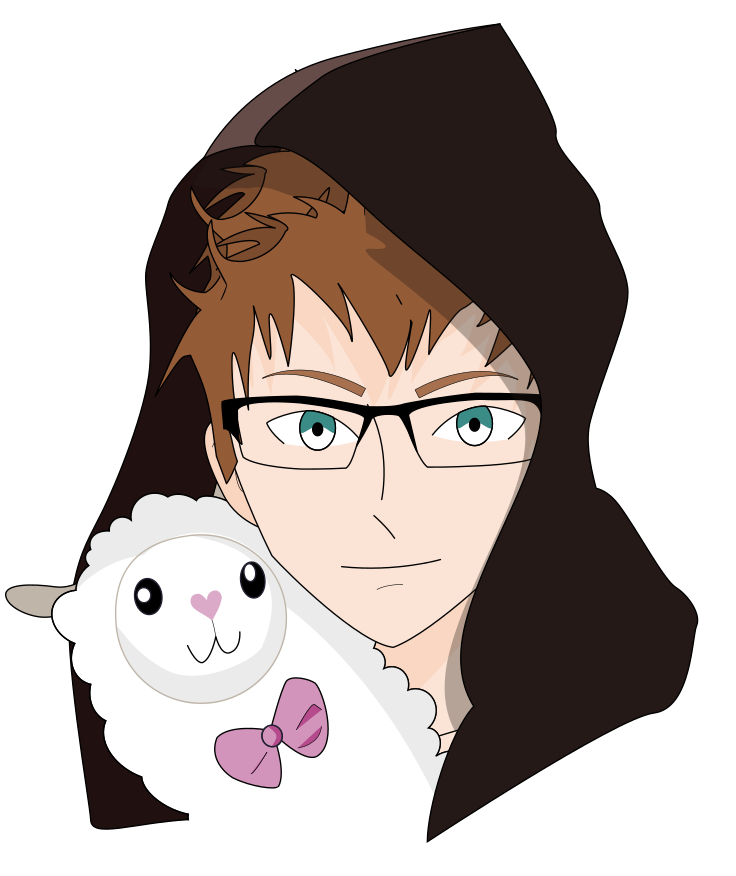
Sam